Microsoft Ajax TextboxWatermark Control
The Microsoft Ajax TextboxWatermark Control is a part of the Microsoft Ajax Toolkit. The Microsoft Ajax Control Toolkit has a rich set of Ajax controls and extenders that can be implemented in your webpages to give you an RIA (Rich Internet Application) style experience.
To use Ajax TextboxWatermark in your websites, you must have the Ajax Control Toolkit installed on your computer. If you don’t have it already, just look for it on Google and download it. Install it by referencing the AjaxToolKit.Dll file in your project.
Implementing the Ajax TextboxWatermark Control
The Ajax TextboxWatermark control is a control extender for the ASP.Net TextBox control. This means that it extends the behavior of the ASP.Net TextBox control. It adds new Ajax functionality to the control.
The watermark concept is simple. It displays some text and styles the TextBox in the specified way if it has no value. In the text you can put in clues to help the user fill the TextBox value correctly. This technique has been used in Windows applications, and is now being used on websites very successfully too.
In this article let’s create a small Page which accepts the User Login ID and Password and use the TextboxWatermark control it.
We’ve dropped in two Textboxes and a button. Here’s how our page looks initially.
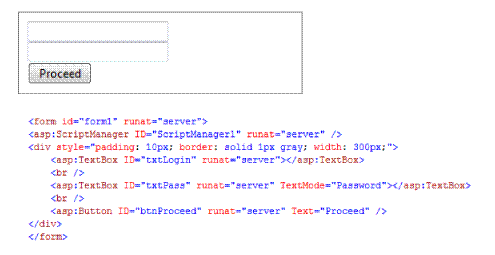
We’ve included the markup too. It’s a simple form with no major styling. Notice that there are no labels specifying which box is for Login ID and which one is for Password. We will use the TextboxWatermark extender to mark them.
Drop in two instances of the TextboxWatermark control extender into the form if you are using Visual Studio 2005. If you have the new Visual Studio 2008, you can simply click on the Textbox in design mode and then on the drop-down menu marker to bring up the context menu for the control. Now click on the ‘Add Extender’ command. You will see a list of Extenders that can be applied to this control. TextboxWatermark extender is one of them. Click to add the extender to your form.
Let’s also examine the actual markup added by ASP.Net to add the extender.
<cc1:TextBoxWatermarkExtender ID="txtLoginExtender" runat="server"
<cc1:TextBoxWatermarkExtender ID="txtLoginExtender" runat="server"
Enabled="True" WatermarkText="Your Login ID" TargetControlID="txtLogin">
</cc1:TextBoxWatermarkExtender>
<br />
<asp:TextBox ID="txtPass" runat="server" TextMode="Password"></asp:TextBox>
<cc1:TextBoxWatermarkExtender ID="txtPassExtender" runat="server"
Enabled="True" WatermarkText="Password" TargetControlID="txtPass">
</cc1:TextBoxWatermarkExtender>
There’s a separate instance of the TextBoxWatermarkExtender for each control, and the TargetControlID property of the extender is set to the name of the control lit extends. Let’s add some text to the watermark.
We have also set the watermark property to the text we want to display in the controls when they don’t have a value. The look is rather simple and the watermark looks as if some text has been already entered.
If you click on any of these Textboxes, the watermark value will disappear. When you click away, it will appear again. The watermark code will not appear if you enter some data in the textbox. This is all the code you need for a functional TextboxWatermark.
Styling the TextboxWatermark Extender
But wait, we can do more than this with our watermark control.
TextboxWatermark allows you to set a CSS class to the property WaterMarkCssClass. Let’s see what happens when we try the CSS below.
<style type="text/css">
.watermark
{
background-color:yellow;
color:Gray;
border:1px solid black;
}
</style>
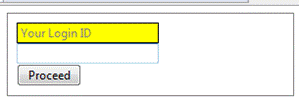
I’ve clicked on the password control to show you that the control reverts to its original design if it is selected or if it has a value.
Here’s our complete code for the TextboxWatermark control along with the styling.
<%@ Page Language="C#" AutoEventWireup="true" CodeFile="Default2.aspx.cs" Inherits="Default2" %>
<%@ Register Assembly="AjaxControlToolkit" Namespace="AjaxControlToolkit" TagPrefix="cc1" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml">
<head runat="server">
<title></title>
<script type="text/javascript">
function pageLoad() {
}
</script>
<style type="text/css">
.watermark
{
background-color:yellow;
color:Gray;
border:1px solid black;
}
</style>
</head>
<body>
<form id="form1" runat="server">
<asp:ScriptManager ID="ScriptManager1" runat="server" />
<div style="padding: 10px; border: solid 1px gray; width: 300px;">
<asp:TextBox ID="txtLogin" runat="server"></asp:TextBox>
<cc1:TextBoxWatermarkExtender ID="txtLoginExtender" runat="server"
Enabled="True" WatermarkText="Your Login ID" WatermarkCssClass="watermark" TargetControlID="txtLogin">
</cc1:TextBoxWatermarkExtender>
<br />
<asp:TextBox ID="txtPass" runat="server" TextMode="Password"></asp:TextBox>
<cc1:TextBoxWatermarkExtender ID="txtPassExtender" runat="server"
Enabled="True" WatermarkText="Password" WatermarkCssClass="watermark" TargetControlID="txtPass">
</cc1:TextBoxWatermarkExtender>
<br />
<asp:Button ID="btnProceed" runat="server" Text="Proceed" />
</div>
</form>
</body>
</html>
The TextboxWatermark’s properties can also be accessed in the Design mode using the Properties toolbox, but I recommend that you make it your habit to work in Source view because if you do that you will get to learn more about how things are implemented in ASP.Net and you can leverage this knowledge in Code-behind.
Working with TextboxWatermark Extender in Code
Talking of Code-behind, did you know that is possible to manipulate your TextboxWatermark extender in code-behind? Though there are not many methods and properties so you can’t do much. But one of the interesting thing that you can do is set the WatermarkText property of the control extender in code. You can set this to a value drawn from the database, or according to a value entered by the user even on some other page. Let’s see how this can be done.
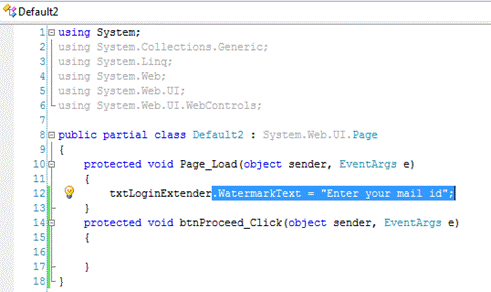
If you run this code you will find that the Login texbox now says ‘Enter your mail id’ instead of ‘Your login ID’ that is because the code in the code-behind over-wrote the property we set in the designer.
Another interesting thing that you can do with the TextboxWatermark extender in code behind is set the TargetControlID property. This can let you select the control to Watermark at runtime. If you are loading the textboxes at runtime, or creating everything from the database then you can use this technique to Watermark your textboxes.
Conclusion
This concludes our tutorial on using the Ajax TextboxWatermark extender in our projects. The TextboxWatermark extender is really an interesting control and can be used very successfully as a UI cue provider.
You must remember though that it is not a replacement for validation, and you must still validate the input for accuracy even if you have told the user what you expect in the textbox. This is called defensive programming. A great technique to ensure that your application always works.
|