How to Use the ASP.Net AJAX FilteredTextBox Extender
Introduction to FilteredTextBox Extender
The
FilteredTextBox Ajax Extender control is a part of the Microsoft Ajax Toolkit
for ASP.Net. The Ajax toolkit is a free, open source Ajax library that can be
used in any ASP.Net project to add rich Ajax functionality to websites. The
Ajax Toolkit can be downloaded free from the Microsoft ASP.Net website.
The
FilteredTextBox Extender is a control extender. This means that it extends the
behavior of an existing ASP.Net control. In this particular case, the control
that is extended is the ASP.Net TextBox. The ASP.Net Textbox does not have any Ajax functionality by itself. The FilteredTextBox extender adds Ajax functionality to the
textbox by injecting Javascript in the client side.
Adding
FilteredTextBox to Your WebPage
To
implement the FIlteredTextBox in your projects add a reference to the MS Ajax
Toolkit DLL. Now all the controls of the ASP.Net Ajax Toolkit will be available
in the toolbar. If you are using Visual Studio 2005, you can drop the
FilteredTextBox control extender in your Source View, and set the ExtendedControl
property to the name of the textbox you want to extend.
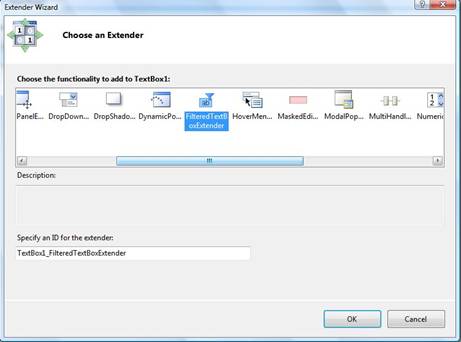
If
you are using Visual Studio 2008, you don’t have to worry about setting the
property. Instead just click on the TextBox control you want to extend; you
will see a arrow at the rightmost edge. Clicking on the arrow will bring a
Context menu with the command ‘Add Extender’. Clicking on Add Extender will
bring a list of Extenders valid for the control. Just click on the
FilteredTextBox extender and it will be added to your Source with all the
properties correctly set for the TextBox control.
Now
your extender control is on the page, and we can modify its behavior.
Working
with the FilteredTextBox
You
can use the FilteredTextBox extender to stop the user from entering garbage
input. You can limit the input in the TextBox to specific characters. If the
user will try to type anything else, nothing will appear.
This
is great to user-proof form Textboxes. For example, if a TextBox is supposed to
accept phone numbers only, this control can make it easier for the user to
enter a correct phone number buy accepting only relevant keypresses like
numbers, ‘-‘, etc.
Examining
the FilterType
The
FilterType property is the most basic property of the extender. It is an
enumeration property with three options: -
1.
LowercaseLetters – The TextBox will accept only lowercase alpahabets, and translate
all uppercase input automatically to lowercase.
2.
Numbers – The TextBox will accept only numeric input.
3.
UppercaseLetters – The TextBox will accept all input only in the Uppercase.
Using
these three properties is quite simple, but the in the practical world you will
need to work with other kinds of inputs too. For everything apart from these
3, there is the Custom FilterType.
When
you set your FilterType to Custom, you will be able to define more freely what
are the characters that you plan to allow, or disallow the user from entering.
You can do that by setting the ValidChars or the InvalidChars property of the
FilteredTextBox Extender.
Let’s
see some code which implements a FilteredTextBox Extender setup to accept a
valid email address.
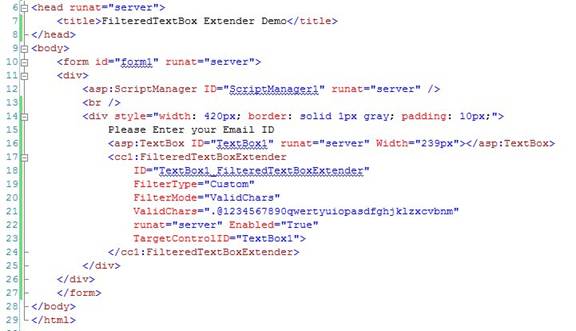
Notic
that we have set the FilterType to Custom, FilterMode to FilterMode to
ValidChars and the ValidChars property to a long string containing all the
characters that are allowed in an email address (all alphabets, numbers and the
‘@.’ symbols.)
When
you set the FilterType to Custom you will also have to set the FilterMode and
the ValidChars or InvalidChars property. The FilterMode property decides
whether you want to set the filter to allow characters or ban characters. You
cannot do both.
If
you want to allow specific characters and ban the rest, set the FilterMode to
ValidChars. If you want to ban some characters and allow the rest, set the
FilterMode to InvalidChars.
Depending
on what you set the FilterMode to, you will also need to set one of the ValidChars
and InvalidChars properties. In the example above we have set ValidChars to all
the characters that are allowed in an email address. If the user tries to enter
any other character than those in the ValidChars, his input will be rejected.
For example, the box will not accept ‘<’, or ‘*’ which are symbols used
often in SQL or Javascript injection attacks.
If
you do the opposite, i.e. set the value of InvalidChars property and set the
FilterMode to ‘InvalidChars’, the TextBox will accept all input except the
characters specified in the InvalidChars. You can use this to ban some special
characters or letters if you are asking the user to enter a code, etc.
Working
in Code
You
can also modify the behavior of the FilteredTextBox Extender in code. This can
be useful if you want to limit the user input based on a database value, or
some value that has been set in your ASP.Net application. Or you can use some
logic to bind the Extender to different TextBoxes. Let’s see a small example: -
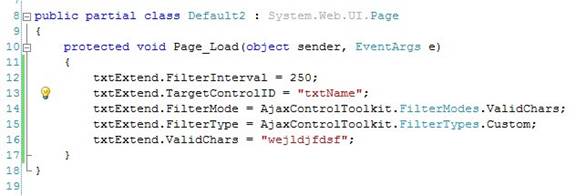
In
this example I have a FilteredTextBox Extender called txtExtend which limits
the input in a Textbox called ‘txtName’ to certain characters. You can set any
of the properties in the Page_Load event, or in a function to get a
FilteredTextBox Extender that works according to your custom code-behind logic.
Also
notice the new property I have introduced here: the FilterInterval. The
FilteredTextBox Extender filters the textbox on timed intervals, and the
FilterInterval property can be used to change the interval time if you wish.
The value defaults to 250ms.
Conclusion
We
have learnt how to use the FilteredTextBox in ASP.Net, and to leverage the
functionality it provides to help our users input correct data. While the
FilteredTextBox will definitely help lessen the incidence of errors in your
application, you must also remember that it is not a guarantee of correct input
and you must still have your code-behind validation in place.
This is because the FilteredTextBox Extender is a Javascript control. If the
users disables Javascript on his browser or has plugins like ‘Noscript’
installed, the Javascript may be stopped from running and the functionality of
the FilteredTextBox Extender will be lost. That is why do not use the extender
to guarantee correct input in critical applications.